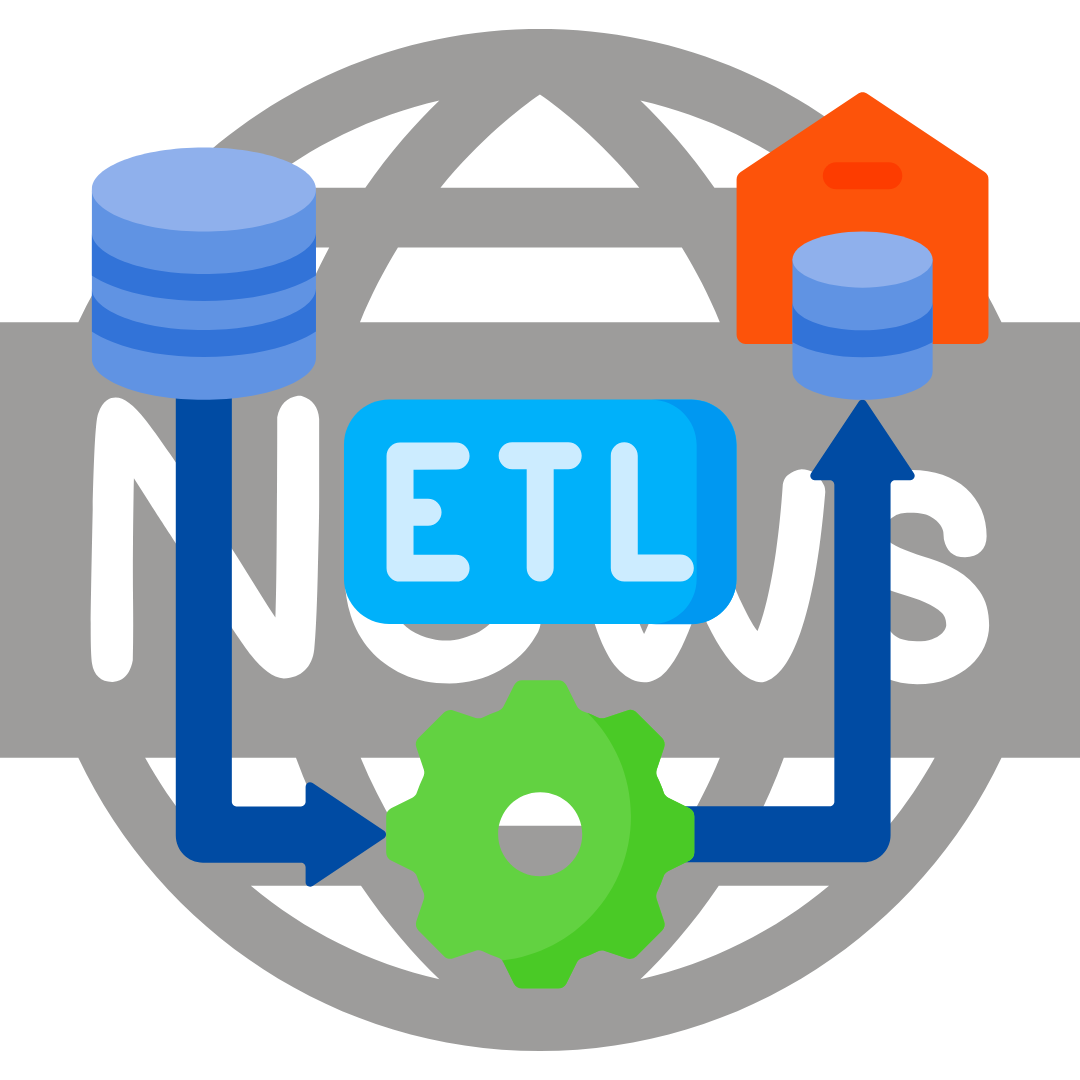
MultiPDF RAGChatBot using Mistral7BInstruct
MultiPDF RAGChatBot using Mistral7BInstruct
import streamlit as st
from streamlit_chat import message
from langchain.chains import ConversationalRetrievalChain
from langchain.embeddings import HuggingFaceEmbeddings
from langchain.llms import LlamaCpp
from langchain.text_splitter import RecursiveCharacterTextSplitter
from langchain.vectorstores import FAISS
from langchain.memory import ConversationBufferMemory
from langchain.document_loaders import PyPDFLoader
import os
os.environ["KMP_DUPLICATE_LIB_OK"]="TRUE"
import tempfile
def initialize_session_state():
if 'history' not in st.session_state:
st.session_state['history'] = []
if 'generated' not in st.session_state:
st.session_state['generated'] = ["Hello! Ask me anything about 🤗"]
if 'past' not in st.session_state:
st.session_state['past'] = ["Hey! 👋"]
def conversation_chat(query, chain, history):
result = chain({"question": query, "chat_history": history})
history.append((query, result["answer"]))
return result["answer"]
def display_chat_history(chain):
reply_container = st.container()
container = st.container()
with container:
with st.form(key='my_form', clear_on_submit=True):
user_input = st.text_input("Question:", placeholder="Ask about your PDF", key='input')
submit_button = st.form_submit_button(label='Send')
if submit_button and user_input:
with st.spinner('Generating response...'):
output = conversation_chat(user_input, chain, st.session_state['history'])
st.session_state['past'].append(user_input)
st.session_state['generated'].append(output)
if st.session_state['generated']:
with reply_container:
for i in range(len(st.session_state['generated'])):
message(st.session_state["past"][i], is_user=True, key=str(i) + '_user', avatar_style="thumbs")
message(st.session_state["generated"][i], key=str(i), avatar_style="fun-emoji")
def create_conversational_chain(vector_store):
# Create llm
llm = LlamaCpp(
streaming = True,
model_path="C:\\Users\\saahi\\Downloads\\mistral-7b-instruct-v0.1.Q4_K_M.gguf",
temperature=0.75,
top_p=1,
verbose=True,
n_ctx=4096
)
memory = ConversationBufferMemory(memory_key="chat_history", return_messages=True)
chain = ConversationalRetrievalChain.from_llm(llm=llm, chain_type='stuff',
retriever=vector_store.as_retriever(search_kwargs={"k": 2}),
memory=memory)
return chain
def main():
# Initialize session state
initialize_session_state()
st.title("Multi-PDF ChatBot using Mistral-7B-Instruct :books:")
# Initialize Streamlit
st.sidebar.title("Document Processing")
uploaded_files = st.sidebar.file_uploader("Upload files", accept_multiple_files=True)
if uploaded_files:
text = []
for file in uploaded_files:
file_extension = os.path.splitext(file.name)[1]
with tempfile.NamedTemporaryFile(delete=False) as temp_file:
temp_file.write(file.read())
temp_file_path = temp_file.name
loader = None
if file_extension == ".pdf":
loader = PyPDFLoader(temp_file_path)
if loader:
text.extend(loader.load())
os.remove(temp_file_path)
text_splitter = RecursiveCharacterTextSplitter(chunk_size=10000, chunk_overlap=20)
text_chunks = text_splitter.split_documents(text)
# Create embeddings
embeddings = HuggingFaceEmbeddings(model_name="sentence-transformers/all-MiniLM-L6-v2",
model_kwargs={'device': 'cpu'})
# Create vector store
vector_store = FAISS.from_documents(text_chunks, embedding=embeddings)
# Create the chain object
chain = create_conversational_chain(vector_store)
display_chat_history(chain)
if __name__ == "__main__":
main()
This Streamlit application demonstrates a Multi-PDF ChatBot powered by Mistral-7B-Instruct language model. The ChatBot allows users to ask questions about the content of uploaded PDF documents and generates conversational response
Imports and Environment Setup:
These imports include various libraries needed for building the chatbot, such as Streamlit for the web interface, Langchain for the conversational chain, and document loaders for processing PDFs.This line sets an environment variable to prevent potential conflicts related to the KMP library.
import streamlit as st
from streamlit_chat import message
from langchain.chains import ConversationalRetrievalChain
from langchain.embeddings import HuggingFaceEmbeddings
from langchain.llms import LlamaCpp
from langchain.text_splitter import RecursiveCharacterTextSplitter
from langchain.vectorstores import FAISS
from langchain.memory import ConversationBufferMemory
from langchain.document_loaders import PyPDFLoader
import os
import tempfile
os.environ["KMP_DUPLICATE_LIB_OK"] = "TRUE"
Session State Initialization:
This function initializes the session state variables to store the conversation history and responses.
def initialize_session_state():
if 'history' not in st.session_state:
st.session_state['history'] = []
if 'generated' not in st.session_state:
st.session_state['generated'] = ["Hello! Ask me anything about 🤗"]
if 'past' not in st.session_state:
st.session_state['past'] = ["Hey! 👋"]
Conversation Handling:
This function processes user queries by using the provided chain and updating the chat history.
def conversation_chat(query, chain, history):
result = chain({"question": query, "chat_history": history})
history.append((query, result["answer"]))
return result["answer"]
Display Chat History:
This function handles the user interface for chat input and displays the conversation history using the streamlit_chat
package
def display_chat_history(chain):
reply_container = st.container()
container = st.container()
with container:
with st.form(key='my_form', clear_on_submit=True):
user_input = st.text_input("Question:", placeholder="Ask about your PDF", key='input')
submit_button = st.form_submit_button(label='Send')
if submit_button and user_input:
with st.spinner('Generating response...'):
output = conversation_chat(user_input, chain, st.session_state['history'])
st.session_state['past'].append(user_input)
st.session_state['generated'].append(output)
if st.session_state['generated']:
with reply_container:
for i in range(len(st.session_state['generated'])):
message(st.session_state["past"][i], is_user=True, key=str(i) + '_user', avatar_style="thumbs")
message(st.session_state["generated"][i], key=str(i), avatar_style="fun-emoji")
Conversational Chain Creation:
This function sets up the conversational chain using the LlamaCpp model and the FAISS vector store for retrieval.
def create_conversational_chain(vector_store):
llm = LlamaCpp(
streaming=True,
model_path="C:\\Users\\saahi\\Downloads\\mistral-7b-instruct-v0.1.Q4_K_M.gguf",
temperature=0.75,
top_p=1,
verbose=True,
n_ctx=4096
)
memory = ConversationBufferMemory(memory_key="chat_history", return_messages=True)
chain = ConversationalRetrievalChain.from_llm(llm=llm, chain_type='stuff',
retriever=vector_store.as_retriever(search_kwargs={"k": 2}),
memory=memory)
return chain
Main Function:
This is the main entry point of the Streamlit application. It initializes the session state, sets up the user interface for file upload, processes the uploaded PDFs, creates text chunks, generates embeddings, builds a vector store, and finally, creates and displays the conversational chain
def main():
initialize_session_state()
st.title("Multi-PDF ChatBot using Mistral-7B-Instruct :books:")
st.sidebar.title("Document Processing")
uploaded_files = st.sidebar.file_uploader("Upload files", accept_multiple_files=True)
if uploaded_files:
text = []
for file in uploaded_files:
file_extension = os.path.splitext(file.name)[1]
with tempfile.NamedTemporaryFile(delete=False) as temp_file:
temp_file.write(file.read())
temp_file_path = temp_file.name
loader = None
if file_extension == ".pdf":
loader = PyPDFLoader(temp_file_path)
if loader:
text.extend(loader.load())
os.remove(temp_file_path)
text_splitter = RecursiveCharacterTextSplitter(chunk_size=10000, chunk_overlap=20)
text_chunks = text_splitter.split_documents(text)
embeddings = HuggingFaceEmbeddings(model_name="sentence-transformers/all-MiniLM-L6-v2",
model_kwargs={'device': 'cpu'})
vector_store = FAISS.from_documents(text_chunks, embedding=embeddings)
chain = create_conversational_chain(vector_store)
display_chat_history(chain)
if __name__ == "__main__":
main()
Components
Streamlit-Chat:
streamlit_chat
is used to display messages in a chat format.- Â
Langchain Components:
ConversationalRetrievalChain: Used to create a retrieval-augmented generation (RAG) pipeline.
HuggingFaceEmbeddings: Generates sentence embeddings for the documents.
LlamaCpp: The language model used to generate responses.
FAISS: A library for efficient similarity search and clustering of dense vectors.
ConversationBufferMemory: Stores the conversation history.
- Â
Document Processing:
PyPDFLoader: Loads and processes PDF documents.
RecursiveCharacterTextSplitter: Splits the text into manageable chunks for processing.
- Â
Embeddings and Vector Store:
Documents are converted into embeddings using a HuggingFace model.
FAISS is used to store and retrieve these embeddings efficiently.
- Â
Conversational Chain:
A conversational chain is created with the LlamaCpp model, embeddings, and a retriever to handle user queries and retrieve relevant information from the uploaded documents.
This application allows users to upload multiple PDF documents and interact with a chatbot that can answer questions based on the content of those documents.
Our Latest Projects
Far far away, behind the word mountains, far from the countries Vokalia and Consonantia
About
An AI Geek and a lifelong learner, who thrives in coding and problem-solving through ML, DL, and LLMs.
Copyright ©2024 All rights reserved.